문제
Given a non-empty array of integers nums, every element appears twice except for one. Find that single one.
You must implement a solution with a linear runtime complexity and use only constant extra space.
int로만 이루어진 nums array에서 2번 이상 반복되지 않는 수를 찾아야 합니다.
예시
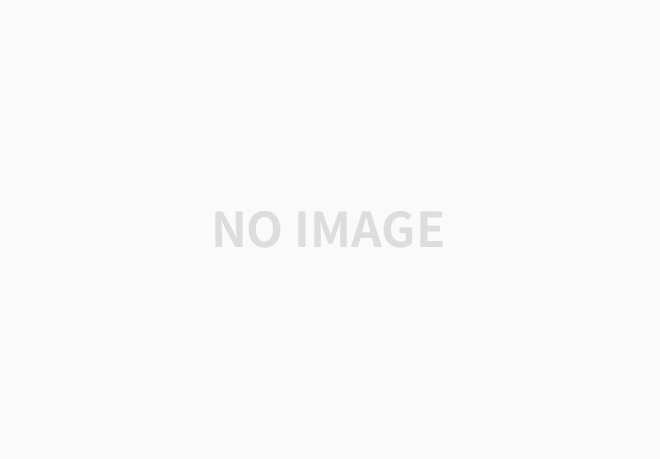
intArray를 input으로 받고 반복되지않은 수를 output으로 내보내야 합니다.
풀이
class Solution {
fun singleNumber(nums: IntArray): Int { //Int를 반환합니다.
val hash_table = HashMap<Int, Int>() //빈 HashMap을 먼저 만듭니다.
for (i in nums) { //nums 의 값들이 들어갑니다.
hash_table[i] = hash_table.getOrDefault(i, 0) + 1
// 만약 IntArray에서 같은 수가 없으면 default값인 0이 리턴되고 거기에 +1을 하게 됩니다.
//getOrDefault 설명
.getOrDefault(Object Key, default Value) 가 들어갑니다.
}
for (i in nums) {
if (hash_table[i] == 1) { // 1로 표현된 값을 찾으면 그게 single number입니다.
return i
}
}
return 0
}
}
이렇게 hasmap을 통하여 간단하게 풀수있습니다.
HashMap을 처음 접하셨다면 저의 Collection정리 포스트를 보고 오시면 도움되실 겁니다.
[Kotlin] Collection 정리 list, set, map 차이 - HashMap, hashmapof, mutableMap, setOf, mutableSetOf,ArrayListof,listof 사용
[Kotlin] Collection 정리 list, set, map 차이 - HashMap, HashMapOf, MutableMap, SetOf, MutableSetOf, ArrayListof, ListOf HashMap, hashmapof, mutableMap, setOf, mutableSetOf, ArrayListof, listof 들의..
underdog11.tistory.com
'개발공부 > LeetCode' 카테고리의 다른 글
[Kotlin] LeetCode: PlusOne 쉬운 풀이 해설 (0) | 2021.06.14 |
---|---|
[Kotlin] LeetCode: Intersection of Two Arrays IISolution 풀이 (0) | 2021.06.11 |
[Kotlin] Array에 반복되는 아이템 삭제하기 - ArrayList distinct - LeetCode: Contains Duplicate - 풀이 과정 (0) | 2021.06.08 |
[Kotlin] LeetCode - Best Time to Buy and Sell Stock IISolution - 풀이과정 (0) | 2021.06.05 |
[Kotlin] String, Int 순서 뒤집기 - LeetCode: Reverse Integer 풀이 (0) | 2021.06.05 |